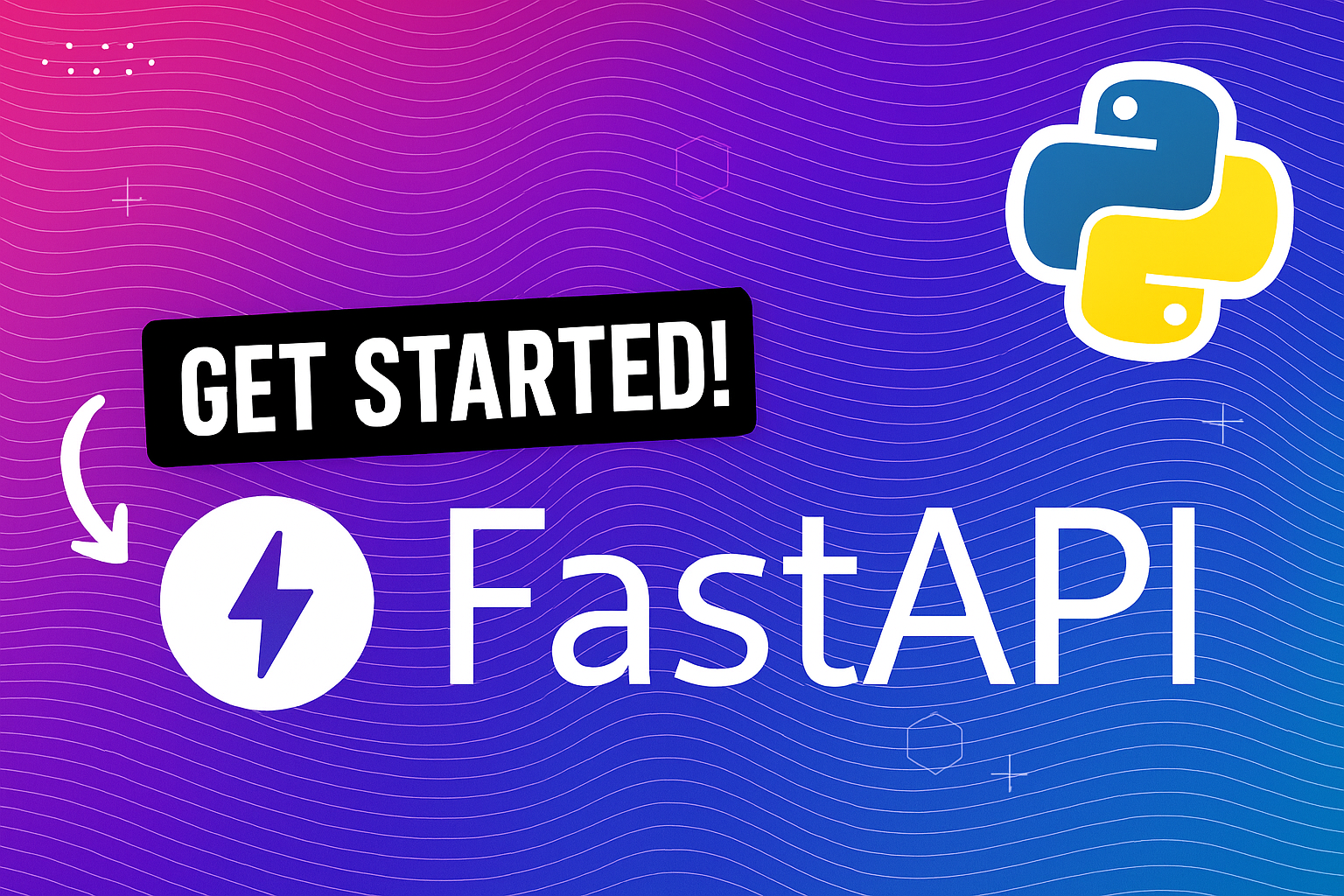
1.Introduction to FastAPI
Why FastAPI?
High Performance:
FastAPI is built on Starlette and Pydantic, supports async features, and its performance is close to Node.js and Go, far surpassing traditional frameworks like Flask and Django.Asynchronous Support:
Native support for async/await, suitable for high-concurrency scenarios.Automatic Documentation:
With simple annotations, FastAPI automatically generates interactive API docs (Swagger UI and ReDoc), making development and testing much easier.
2.Project Setup
1 | pip install fastapi uvicorn |
3.Creating Your First API
1 | from fastapi import FastAPI |
4.Adding Routes and Pydantic Models
1 | from pydantic import BaseModel |
5.A Simple FastAPI Application Example
1 | from fastapi import FastAPI |
The following image shows the interactive API documentation (Swagger UI) automatically generated by FastAPI, where developers can directly test the endpoints.
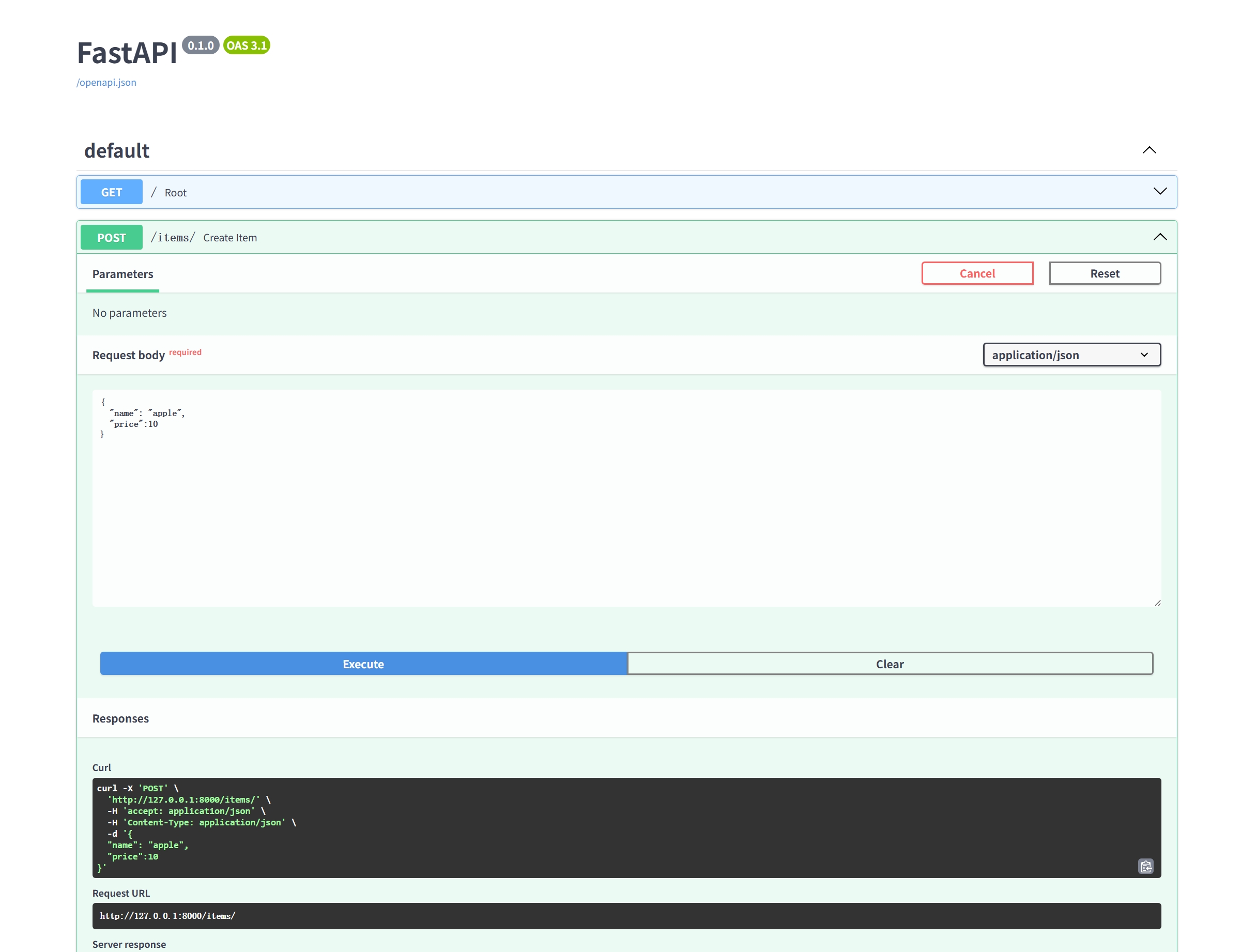